Button の装飾について
ButtonのCSSを用いた装飾方法を解説します。
Button
外部ファイルのCSSをロードしてButtonを装飾するサンプルプログラムです。
- 1 :
2 :
3 :
4 :
5 :
6 :
7 :
8 :
9 :
10 :
11 :
12 :
13 :
14 :
15 :
16 :
17 :
18 :
19 :
20 :
21 :
22 :
23 :
24 :
25 :
26 :
27 :
28 :
29 :
30 :
31 :
32 :
33 :
34 :
35 :
36 :
37 :
38 :
39 :
40 :
41 :
42 :
43 : -
import javafx.application.Application; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.layout.StackPane; import javafx.stage.Stage; public class JavaFX_ButtonCSS extends Application{ public static void main(String... args){ Application.launch(args); } public void start(Stage stage) throws Exception { // Buttonを作成 Button button = new Button("Button Style Example"); // ButtonにStyleClassを設定 button . getStyleClass() . add("Button_CSS"); StackPane stack = new StackPane( ); stack . setAlignment( Pos . CENTER ); stack . getChildren( ) . addAll( button ); // Sceneを作成 Scene scene = new Scene( stack ); // StyleSheetsの設定 scene . getStylesheets() . add( getClass() . getResource("css/javaFX.css") . toExternalForm() ); stage . setTitle("Button Style Example"); stage . setWidth( 300 ); stage . setHeight( 300 ); stage . setScene( scene ); stage . show(); } }
【 サンプルソース No.1 JavaFX_ButtonCSS 】
ポイント
Buttonに設定するStyleのクラスを設定しているコード
- 21 :
22 : -
// ButtonにStyleClassを設定 button . getStyleClass() . add("Button_CSS");
Sceneに対してスタイルシートを読み込む
- 32 :
33 : -
// StyleSheetsの設定 scene . getStylesheets() . add( getClass() . getResource("css/javaFX.css") . toExternalForm() );
javaFX.css
javaFX.cssの内容です。
- 1 :
2 :
3 :
4 :
5 :
6 :
7 :
8 :
9 :
10 :
11 :
12 :
13 :
14 :
15 :
16 :
17 :
18 :
19 :
20 :
21 :
22 :
23 :
24 :
25 :
26 :
27 :
28 :
29 :
30 : -
.Button_CSS { -fx-pref-width : 200; /* 横幅 */ -fx-pref-height : 100; /* 高さ */ -fx-background-color : gray; /* 背景色 */ -fx-alignment : center; /* 位置 */ -fx-text-fill : white; /* 文字色 */ -fx-underline : true; /* 下線 */ -fx-border-style : solid outside; /* 枠線のスタイル */ -fx-border-color : skyblue; /* 枠線の色 */ -fx-border-width : 1; /* 枠線の太さ */ -fx-font-family : sans-serif; /* フォント */ -fx-font-size : 12pt ; /* フォントサイズ */ -fx-font-style : italic; /* フォントスタイル */ -fx-font-weight : bold; /* フォントの太さ */ -fx-background-radius : 50px; /* 背景の角丸み */ -fx-border-radius : 50px; /* 枠線の角丸み */ } .Button_CSS:hover { -fx-text-fill : red; /* 文字色 */ -fx-border-color : red; /* 枠線の色 */ } .Button_CSS:pressed { -fx-background-color : gray; /* 背景色 */ -fx-border-width : 1; /* 枠線の太さ */ -fx-text-fill : silver; /* 文字色 */ -fx-border-color : black; /* 枠線の色 */ -fx-border-style : solid inside; /* 枠線のスタイル */ }
各スタイルの詳細はこちらをご覧ください。
- 01.背景色[ -fx-background-color ]2020/07/23
- 02.文字色[ -fx-text-fill ]2020/07/23
- 03.フォント[ -fx-font ]2020/07/23
- [ -fx-font-family ]2020/07/23
- [ -fx-font-size ]2020/07/23
- [ -fx-font-style ]2020/07/23
- [ -fx-font-weight ]2020/07/23
- 04.枠線の色[ -fx-border-color ]2020/07/23
- 05.枠線の幅[ -fx-border-width ]2020/07/23
- 06.枠線のスタイル[ -fx-border-style ]2020/07/23
- 07.コントールサイズ[ -fx-prefWidht,-fx-prefHeight ]2020/08/13
- [ -fx-maxWidht,-fx-maxHeight ]2020/08/13
- 08.テキスト位置[ -fx-alignment ]2020/08/14
- 09.アンダーライン[ -fx-underline ]2020/08/14
- 10.背景 角の丸み[ -fx-background-radius ]2020/08/15
- 11.枠線 角の丸み[ -fx-border-radius ]2020/08/15
実行結果
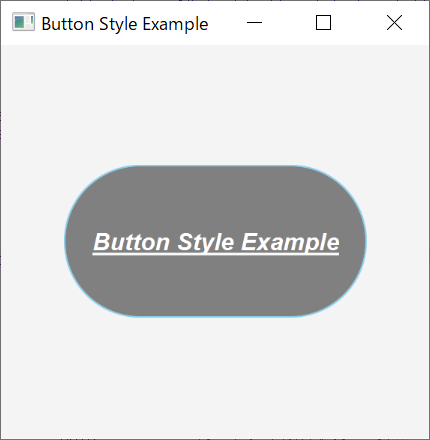