CheckBoxについての考察
通常、CheckBoxは「チェックされている」「チェックされていない」の二択で使用しますが、さらに「未定義」という状態を含めた三択で使用することができます。「未定義」という表現が少し分かりづらく感じたので、本サイトでは、当該CheckBoxの項目は「対象外の場合」という意味でとらえています。CheckBoxで選択する項目自体が、対象外の場合などに使用するのではないかと思います。
使用方法としては、まずプロパティindeterminateプロパティの内容で、当該CheckBoxが「対象」又は「対象外」の判断をします。その後に、CheckBoxが「対象」の場合に限り、チェック有り/無しの判定をします。
1 | 対象外 (indeterminate=true) |
- |
![]() |
---|---|---|---|
2 | 対象 (indeterminate=false) |
チェック有り (Selected=true) |
![]() |
3 | チェック無し (Selected=false) |
![]() |
CheckBoxを対象外(未定義)にする
当該CheckBoxを対象外(未定義)にするには、setIndeterminateメソッドを使用して、プロパティindeterminateをtrueに設定します。
- 1 :
2 :
3 :
4 :
5 :
6 :
7 :
8 :
9 :
10 :
11 :
12 :
13 :
14 :
15 :
16 :
17 :
18 :
19 :
20 :
21 :
22 :
23 :
24 :
25 :
26 :
27 :
28 :
29 :
30 :
31 :
32 :
33 :
34 :
35 :
36 :
37 :
38 : -
import javafx.application.Application; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.CheckBox; import javafx.scene.layout.VBox; import javafx.stage.Stage; public class JavaFX_CheckBox4 extends Application { public static void main(String ... args){ Application . launch(args); } @Override public void start(Stage stage) throws Exception { // チェックボックスの作成 CheckBox checkBox1 = new CheckBox( "CheckBox" ); checkBox1.setIndeterminate(true); // レイアウト VBox vbox = new VBox(); vbox . setAlignment( Pos . CENTER); vbox . getChildren() . addAll( checkBox1 ); // sceanの配置 Scene scene = new Scene( vbox ); stage . setTitle( "CheckBox" ); stage . setWidth( 300 ); stage . setHeight( 200 ); stage . setScene( scene ); stage . show(); } }
【 サンプルソース No.1 CheckBoxを対象外にする 】
実行結果
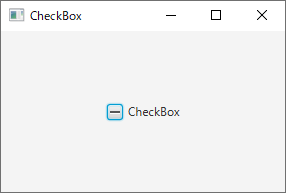
- 21 :
-
checkBox1.setIndeterminate(true);
CheckBoxの入力値を、checked、unchecked、undefinedの循環切替に設定する
CheckBoxの状態を「チェック有り」「チェック無し」「対象外」の循環切替にすることが可能です。 setAllowIndeterminateメソッドを使用して、allowIndeterminateをtrueに設定することで、CheckBoxの入力値をchecked、unchecked、undefinedの循環切り替えに設定することが可能です。
- 1 :
2 :
3 :
4 :
5 :
6 :
7 :
8 :
9 :
10 :
11 :
12 :
13 :
14 :
15 :
16 :
17 :
18 :
19 :
20 :
21 :
22 :
23 :
24 :
25 :
26 :
27 :
28 :
29 :
30 :
31 :
32 :
33 :
34 :
35 :
36 :
37 :
38 : -
import javafx.application.Application; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.CheckBox; import javafx.scene.layout.VBox; import javafx.stage.Stage; public class JavaFX_CheckBox5 extends Application { public static void main(String ... args){ Application . launch(args); } @Override public void start(Stage stage) throws Exception { // チェックボックスの作成 CheckBox checkBox1 = new CheckBox( "CheckBox" ); checkBox1.setAllowIndeterminate(true); // レイアウト VBox vbox = new VBox(); vbox . setAlignment( Pos . CENTER); vbox . getChildren() . addAll( checkBox1 ); // sceanの配置 Scene scene = new Scene( vbox ); stage . setTitle( "CheckBox" ); stage . setWidth( 300 ); stage . setHeight( 200 ); stage . setScene( scene ); stage . show(); } }
【 サンプルソース No.1 CheckBoxを循環切替に設定する 】
実行結果
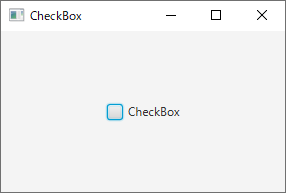
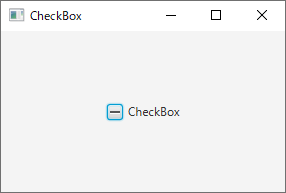
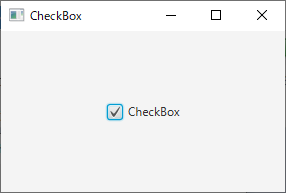
- 21 :
-
checkBox1.setAllowIndeterminate(true);
